RecyclerView Part 2: Android RecyclerView Adapter
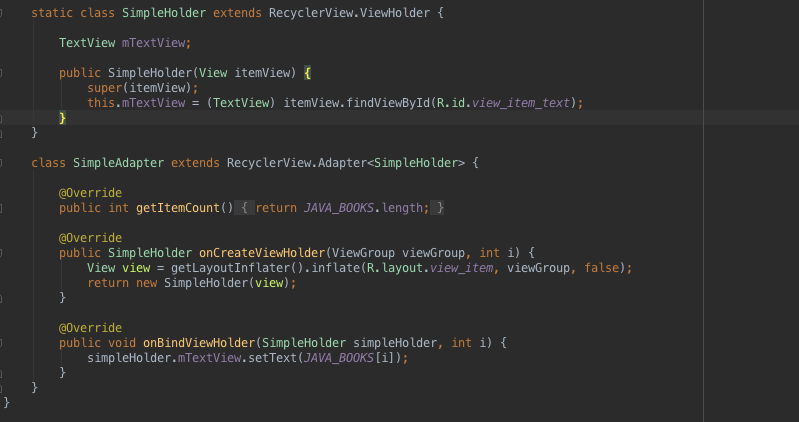
This is the second post in our Android RecyclerView series. In previous post, you have find a very basic example about the RecyclerView
in Android development. In this post, we will go deeper about one important element with RecyclerView: the Adapter.
Please refer to the source code which has been posted from previous post at GitHub. We will use it as reference for this post, as well as all other posts in this serie.
Android RecyclerView Adapter
First of all, the Adapter class of a RecyclerView does not extends from BaseAdapter
like ListView and so on. It inherits directly from Object. So you can imagine now the RecyclerView works mostly different from ListView, because:
- It does not place views on their positions on screen
- It does not animate views either
- Except scrolling, it does not handle any touch events
Sound weird, right? But as its name, RecyclerView
means the only thing it does, is recycling the view. While the Adapter is basically the binding between RecyclerView data and its presentation view, the RecyclerView itself displays internal views using LayoutManager
, it animates elements using ItemAnimator
class, and finally, it leaves touch events like tap, click for us to handle ourself.
Basic Adapter
When we provide adapter to our RecyclerView, we usually have to implement the following methods:
- getItemCount(): similar to ListView adapter, we need to tell the RecyclerView know about total items inside our data block
- [onCreateViewHolder](https://developer.android.com/reference/android/support/v7/widget/RecyclerView.Adapter.html#onCreateViewHolder(android.view.ViewGroup, int))(ViewGroup parent, int viewType): many developers understand second paramater is position of item. Actually it is the view type. This field is useful when our RecyclerView and our Adapter have more than one type of view item. For example, we want some rows have layout A, while others use layout B instead. That’s where viewType comes into play. In normal case, we usually get the layout inflater from activity context to construct view from xml files, or create the view programmatically and construct view holder object here. We will talk about the holder later.
- [onBindViewHolder](https://developer.android.com/reference/android/support/v7/widget/RecyclerView.Adapter.html#onBindViewHolder(VH, int))(VH holder, int position): you can see the method
getView()
from a normal adapter of ListView/GridView has been clearly splitted to two methods, the construction is done by the method above, while the presentation is done by this method. Based on position, you give views inside the holder, which you have created before, the necessary properties like text content, image, etc.
The Holder Pattern
Back in ListView era, we usually hear about holder pattern. This pattern is used to avoid creating new view each time the row have been refreshed. We use a temporary holder class to holder all view related properties so we can avoid calling findViewById which cost our CPU cycles as list can be scrolled many fast. Now Google has give us official support for this pattern.
By making the holder generics, developers are free to create their own holder. Each holder which attached to the adapter has its own root view, which is the view used to create the holder:
static class SimpleHolder extends RecyclerView.ViewHolder {
TextView mTextView;
public SimpleHolder(View itemView) {
super(itemView);
this.mTextView = (TextView) itemView.findViewById(R.id.view_item_text);
}
}
class SimpleAdapter extends RecyclerView.Adapter < SimpleHolder > {
@Override public int getItemCount() {
return JAVA_BOOKS.length;
}
@Override public SimpleHolder onCreateViewHolder(ViewGroup viewGroup, int i) {
View view = getLayoutInflater().inflate(R.layout.view_item, viewGroup, false);
return new SimpleHolder(view);
}
@Override public void onBindViewHolder(SimpleHolder simpleHolder, int i) {
simpleHolder.mTextView.setText(JAVA_BOOKS[i]);
}
}
In next post, we will talk about one important thing which RecyclerView does not do for us: the click handling on item.