Using Video as Background for iOS App
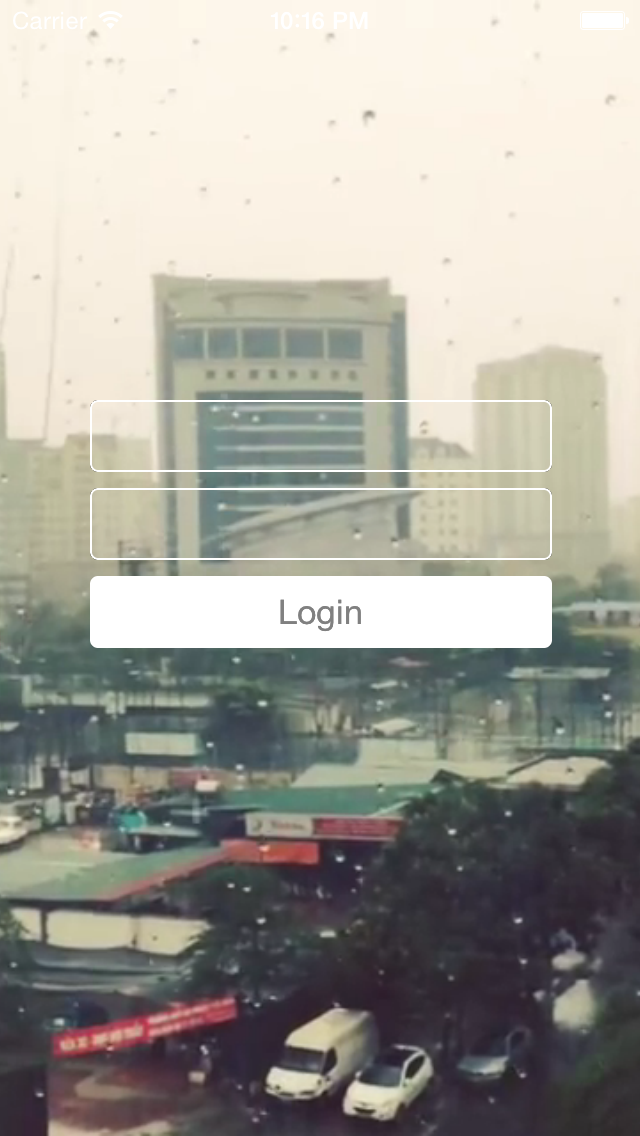
You should be familiar with many login screen in mobile app, boring username field, then a password field, and some button for log user in, forgot password, etc. You can try add some icons, fancy color or animation to field with validation and social login, but you should admit that it is still boring.
You want another look and feel for your login screen, so, I will tell you, how about this?
Yes, we are going to add a video as background for your login screen.
Start with project from Parallax Posts, we add one more view controller with a storyboard ID:
[![ViewController_WithIom/content/images/2015/07/ViewController_WithID.png)
Prepare Video
Our video should be in .mp4 format (Apple standard video) to be easily read from app, and do not add a video which size more than 10MB, it will blow up your app. The available video should be less than 5MB in size, as it should be a loopable video, which mean when it plays repealy, user can not distinguish between start and end of the video. I will take a my recent video in Instagram as example, it’s about more than 800KB:
Có tí gió, có tí mưa #rain by @luceefer
Drag the video into XCode:
Create Video Background
First, we create a new controller for our screen:
[
Declare new class:
import UIKit class LoginController: UIViewController { }
Back to previous created view controller inside storyboard, we assign newly created controller to it custom class:
We will skip parallax screen as previously done before, we will instantiate and present new controller right away:
override func viewDidAppear(animated: Bool) {
let vc = self.storyboard?.instantiateViewControllerWithIdentifier("LoginScreen") as! UIViewController
self.presentViewController(vc, animated: true, completion: nil)
}
Setup VideoPlayer
Go to new controller, we are going to use AVFoundation to play video:
import AVFoundation
First, identify path of our video:
let path = NSBundle.mainBundle().pathForResource("BackgroundVideo", ofType: "mp4")
Create a player based on this path:
let player = AVPlayer(URL: NSURL(fileURLWithPath: path!))
Create a layer to present this player:
let playerLayer = AVPlayerLayer(player: player)
Set frame, repeat count and scale mode for this layer:
playerLayer.frame = self.view.frame playerLayer.videoGravity = AVLayerVideoGravityResizeAspectFill
Add view from this layer to our view:
self.view.layer.addSublayer(playerLayer)
Finally, play the video:
player.seekToTime(kCMTimeZero) player.play()
You can run project for now. You should see the video is playing fullscreen. We just add some elements:
[
Repeat
You can notice that our video plays just once. AVPlayer
provides a notification which we can use NSNotificationCenter
to listen when the player has reached its end. First, we change our declaration to var instead of let:
var player: AVPlayer?
Then, listen to notification:
NSNotificationCenter.defaultCenter().addObserver(self, selector: "playerItemDidReachEnd", name: AVPlayerItemDidPlayToEndTimeNotification, object: player!.currentItem)
In notification callback, we simply seek back to start position:
func playerItemDidReachEnd() {
player!.seekToTime(kCMTimeZero)
}
Then, our video is ready to loop!
Parallax
You remember the era when you just upgrade from iOS6 to iOS7, do you? The home screen of your iPhone just moving and shaking with your hand, that was something Apple called parallax or motion. We can add a similar effect to our login view by some lines of code.
First, we create a vertical UIMotionEffect:
let verticalMotionEffect = UIInterpolatingMotionEffect(keyPath: "center.y", type: .TiltAlongVerticalAxis)
verticalMotionEffect.minimumRelativeValue = -10
verticalMotionEffect.maximumRelativeValue = 10
Another horizontal one:
let horizontalMotionEffect = UIInterpolatingMotionEffect(keyPath: "center.x", type: .TiltAlongHorizontalAxis)
horizontalMotionEffect.minimumRelativeValue = -10
horizontalMotionEffect.maximumRelativeValue = 10
Now, create a group for both effects, and assign it to our root view:
let group = UIMotionEffectGroup()
group.motionEffects = [horizontalMotionEffect, verticalMotionEffect]
self.view.addMotionEffect(group)
You can run project, and notice that the video will move alongside with device. Note that you have to use a REAL device to see this effect, and do not forget turn off General -> Accessibility -> Reduce Motion.
Yeah, that’s all about another way to make your view more awesome, you can find source code for this post at tag video-view in GitHub. Thank you!