Add Facebook Login into Your App
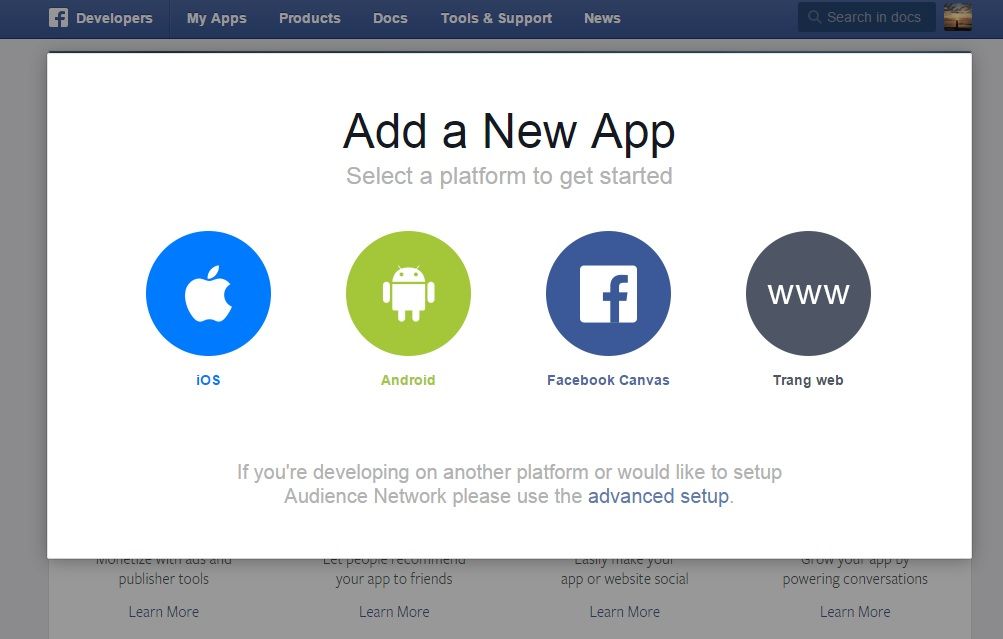
Prerequisites
- Android Studio
- Facebook account
Register your app
When use Facebook SDK, we must be registered with Facebook. Login to Facebook Developers Website and create a new app.
Select Android on pop-up.
Enter name for your new app and select “Create New Facebook App Id”
Enter “Package Name” and “Default Activity Class Name”. Select “Next”
[![android_add_facebookom/content/images/2015/07/android_add_facebook_login_4.jpg)
In next form, enter key hashes. There are two way to get key hashes.
- Open a terminal window and run the key tool command to generate a key has using the bebug keystore located at
~/.android/debug.keystore
. This is what the command should look like.
keytool -exportcert -alias androiddebugkey -keystore ~/.android/debug.keystore | openssl sha1 -binary | openssl base64
- You can get key hashes with source code:
try {
PackageInfo info = getPackageManager().getPackageInfo(" net.awpspace.demo", PackageManager.GET_SIGNATURES);
for (Signature signature: info.signatures) {
MessageDigest md = MessageDigest.getInstance("SHA");
md.update(signature.toByteArray());
Log.d("KeyHash:", Base64.encodeToString(md.digest(), Base64.DEFAULT));
}
} catch (NameNotFoundException e) {} catch (NoSuchAlgorithmException e) {}
Add Facebook SDK to your project
Add this to Mudule-level /app/build.gradle
before dependencies:
repositories { mavenCentral() }
Add the compile dependency with the lastest version of the Facebook SDK in the build.gradle file:
dependencies { compile 'com.facebook.android:facebook-android-sdk:4.1.0' }
Create an Activity
Step 1: Define the layout:
Create a new layout file name main_activity.xml
in res/layout
. In this layout:
- LoginButton to allow the user to login to Facebook.
- TextView to display the result of the lastest login attempt.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/info"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:textSize="18sp" />
<com.facebook.login.widget.LoginButton
android:id="@+id/login_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true" />
</RelativeLayout>
Step 2: Create the Class
Create a new java class that extends Activity and name it MainActivity.java
. Declare the widget you defined in the activity’s layout as fields of this class.
private TextView info;
private LoginButton loginButton;
Declare a CallbackManager
as another field.
private CallbackManager callbackManager;
The SDK needs to be initialized before using any of its methods. You can do so by calling sdkInitialize
and passing the application’s context. Add the following code to the onCreate
method of your Activity
:
FacebookSdk.sdkInitialize(getApplicationContext());
Next, initialize your instance of CallbackManager
using the CallbackManager.Factory.create
method.
callbackManager = CallbackManager.Factory.create();
Call setContent
to set the layout defined in the previous step as the layout of this Activity and then use findViewById
to initialize the widgets.
setContentView(R.layout.main_activity);
info = (TextView) findViewById(R.id.info);
loginButton = (LoginButton) findViewById(R.id.login_button);
Register the custom callback, use the registerCallback
method. The code to create and register the callback should look like:
loginButton.registerCallback(callbackManager, new FacebookCallback < LoginResult > () {
@Override public void onSuccess(LoginResult loginResult) {}
@Override public void onCancel() {}
@Override public void onError(FacebookException e) {}
});
Add the following code to the onSuccess
method:
info.setText( "User ID: " + loginResult.getAccessToken().getUserId() + "\n" + "Auth Token: " + loginResult.getAccessToken().getToken() );
Add the following code to the onCancel
method:
info.setText("Login attempt canceled.");
Add the following code to the onError
method:
info.setText("Login attempt failed.");
Tapping the login btton starts off a new Activity, which returns a result. To receive and handle the result, override the onActivityResult
method of you Activity and pass its parameters to the onActivityResult
method of CallbackManager
.
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) {
callbackManager.onActivityResult(requestCode, resultCode, data);
}
Add the Facebook Application ID
The application ID you received when you registered your app should be added as a string in your project’s res/value/strings.xml
. Fro this tutorial, call the string facebook_app_id
.
<string name="facebook_app_id">123456908761030</string>
Edit the Manifest
Define your Activity in the AndroidManifest.xml
.
<activity android:name="com.hathy.fblogin.MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
Add the application ID as meta-data.
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id" />
Define FacebookActivity.
<activity
android:name="com.facebook.FacebookActivity"
android:configChanges= "keyboard|keyboardHidden|screenLayout|screenSize|orientation"
android:theme="@android:style/Theme.Translucent.NoTitleBar"
android:label="@string/app_name" />
Add permission for your app.
<uses-permission android:name="android.permission.INTERNET"/>
Build and Run
Your app is now complete. When you build it and deploy it on your Android device you will see the Facebook login button.
[![android_add_facebookom/content/images/2015/07/android_add_facebook_login_6.jpg)
Conclusion
In this article, you learned how to use the Facebook SDK to add Facebook Login to your Android app. To learn more about Facebook Login, you can go throught the reference for the Facebook SDK for Android.