Barcode detection with the Mobile Vision API
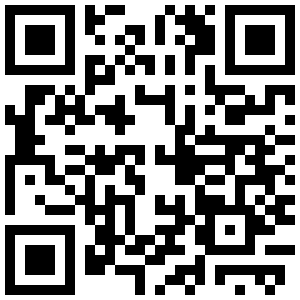
Recently Google release GooglePlayServices 7.8 to build better apps. You can get new highlights in this release at here. And now in this article I will show you how to make simple sample for barcode detection using Mobile Vision Api that is a highlight in new release of GooglePlayService.
Prerequisites
Before beginning, check that you have all the necessary pre-requisites. These include:
- Android Studio
- Android device or Emulator that runs Android 4.2.2 or later
- The latest version of the Android SDK including the SDK tools component
- The GooglePlayServices SDK
Create new project
In Android Studion, you create new project with name is BarcodeDetect.
[![barcode_detection_neom/content/images/2015/08/barcode_detection_new_project.jpg)
Update GooglePlayServices
Open Android SDK Manager then find the entry for GooglePlayServices and make sure you have version 26 or higher:
[![update_google_play_som/content/images/2015/08/update_google_play_service.jpg)
Configure build.gradle
To use GooglePlayServices you must configure build.gradle file to add GooglePlayServices to your module. Open build.gradle
file
[
and add a dependency like below:
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
compile 'com.android.support:appcompat-v7:22.2.1'
compile 'com.google.android.gms:play-services:7.8+'
}
Adding a UI to your app
Open activity_main.xml
file and make UI like below
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity">
<LinearLayout android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView android:id="@+id/txtContent"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="" />
<Button android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:text="Process" />
<ImageView android:id="@+id/imgview"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
</RelativeLayout>
To keep simple things, for this sample, you’re just going to process an image that is already present in your app.
Coding your application
The first step, you must load image to ImageView.
ImageView myImageView = (ImageView) findViewById(R.id.imgview);
final Bitmap myBitmap = BitmapFactory.decodeResource(getApplicationContext().getResources(), R.drawable.barcode);
myImageView.setImageBitmap(myBitmap);
The second step, you will handle button event
Button btn = (Button) findViewById(R.id.button);
btn.setOnClickListener(new View.OnClickListener() {
@Override public void onClick(View v) {}
});
In onClick
method, I will show you how to detect and decode barcode. Detect the barcode is pretty straightforward, it creates a new BarcodeDetector, using a builder. It then creates a frame from the bitmap, and passes it to the detector. This return a SparseArray
of barcodes.
//Detect the barcode
BarcodeDetector detector = new BarcodeDetector.Builder(getApplicationContext()).setBarcodeFormats(Barcode.DATA_MATRIX | Barcode.QR_CODE).build();
Frame frame = new Frame.Builder().setBitmap(myBitmap).build();
SparseArray < Barcode > barcodes = detector.detect(frame);
Decode the barcode. Because for this sample, we have 1 and only 1 barcode, then the size of SparseArray
is 1.
//Decode the barcode
Barcode thisCode = barcodes.valueAt(0);
TextView txtView = (TextView) findViewById(R.id.txtContent);
txtView.setText(thisCode.rawValue);
Done. Now all you have to do is run the app. You can see the data encoded in the QR Code.
[![barcode_detection_reom/content/images/2015/08/barcode_detection_result.png)