Extract Prominent Colors For Android Apps With Palette
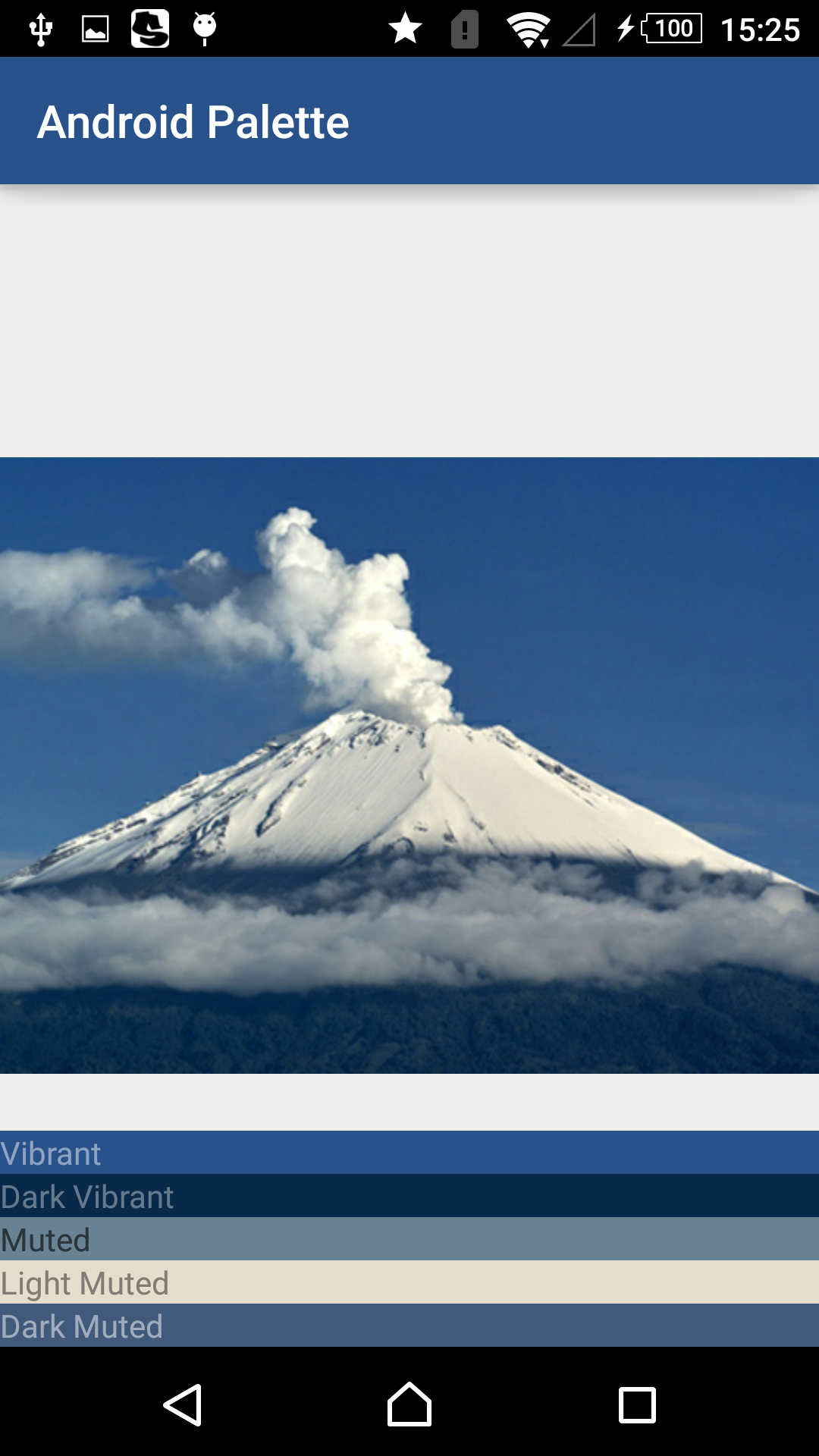
In Android Lollipop, a new defining features of material design is the use of color.
You can get more infomation in here: Material Design Color
In this article, I will show you how to extract prominent colors from a bitmap for customizing UI. I also have a sample for you which use Palette object to get color profile of bitmap.
[![android_palette_coloom/content/images/2015/07/android_palette_color.png)In the sample, I get vibrant color from a bitmap then change background color for ActionBar with this.
Import the Palette
To get started, you need import the palatte support library into your project.
compile 'com.android.support:palette-v7:22.2.0'
Add to the project’s build.gradle file and run gradle sync. With Palette, you can get different color profiles from the image.
- Vibrant
- Vibrant Dark
- Vibrant Light
- Muted
- Muted Dark
- Muted Light
Instances are created with a Palette.Builder which supports several options to tweak the generated Palette.
Generation should always be completed on a background thread, ideally the one in which you load your image on. Palette.Builder supports both synchronous and asynchronous generation:
// Synchronous
Palette p = Palette.from(bitmap).generate(); // Asynchronous
Palette.from(bitmap).generate(new PaletteAsyncListener() {
public void onGenerated(Palette p) { // Use generated instance } });
Swatches
Swatch objects represents a color swatch generated from an image’s palette. The RGB color can be retrieved by calling getRgb().
Each Palette has a set of six predefined color profiles, I use six TextViews to show this.
mVibrantTextView = (TextView) findViewById(R.id.vibrant);
mLightVibrantTextView = (TextView) findViewById(R.id.light_vibrant);
mDarkVibrantTextView = (TextView) findViewById(R.id.dark_vibrant);
mMutedTextView = (TextView) findViewById(R.id.muted);
mLightMutedTextView = (TextView) findViewById(R.id.light_muted);
mDarkMutedTextView = (TextView) findViewById(R.id.dark_muted);
Palette.from(bitmap).generate(new Palette.PaletteAsyncListener() {
@Override public void onGenerated(Palette palette) {
mActionBar.setBackgroundDrawable(newColorDrawable(palette.getVibrantSwatch().getRgb()));
setViewSwatch(mVibrantTextView, palette.getVibrantSwatch());
setViewSwatch(mLightVibrantTextView, palette.getLightVibrantSwatch());
setViewSwatch(mDarkVibrantTextView, palette.getDarkVibrantSwatch());
setViewSwatch(mMutedTextView, palette.getMutedSwatch());
setViewSwatch(mLightMutedTextView, palette.getLightMutedSwatch());
setViewSwatch(mDarkMutedTextView, palette.getDarkMutedSwatch());
}
});
private void setViewSwatch(TextView view, Palette.Swatch swatch) {
if (swatch != null) {
view.setTextColor(swatch.getTitleTextColor());
view.setBackgroundColor(swatch.getRgb());
view.setVisibility(View.VISIBLE);
} else {
view.setVisibility(View.GONE);
}
}
In additional to the standard profile Swatch objects, each Palette contains a list of general Swatch objects generated from the bitmap. These can retrieved from the Palette as a List by using the getSwatches() method.
for (Palette.Swatch swatch : palette.getSwatches()) {
//do sth with swatch
}
Conclusion
In this article, you have learned about the Palette and how to extract swatches of color from bitmap. You can get complete source code in here: Android Palette (module: androidpalette)