Implement ListViewHeader with Parallax Scrolling
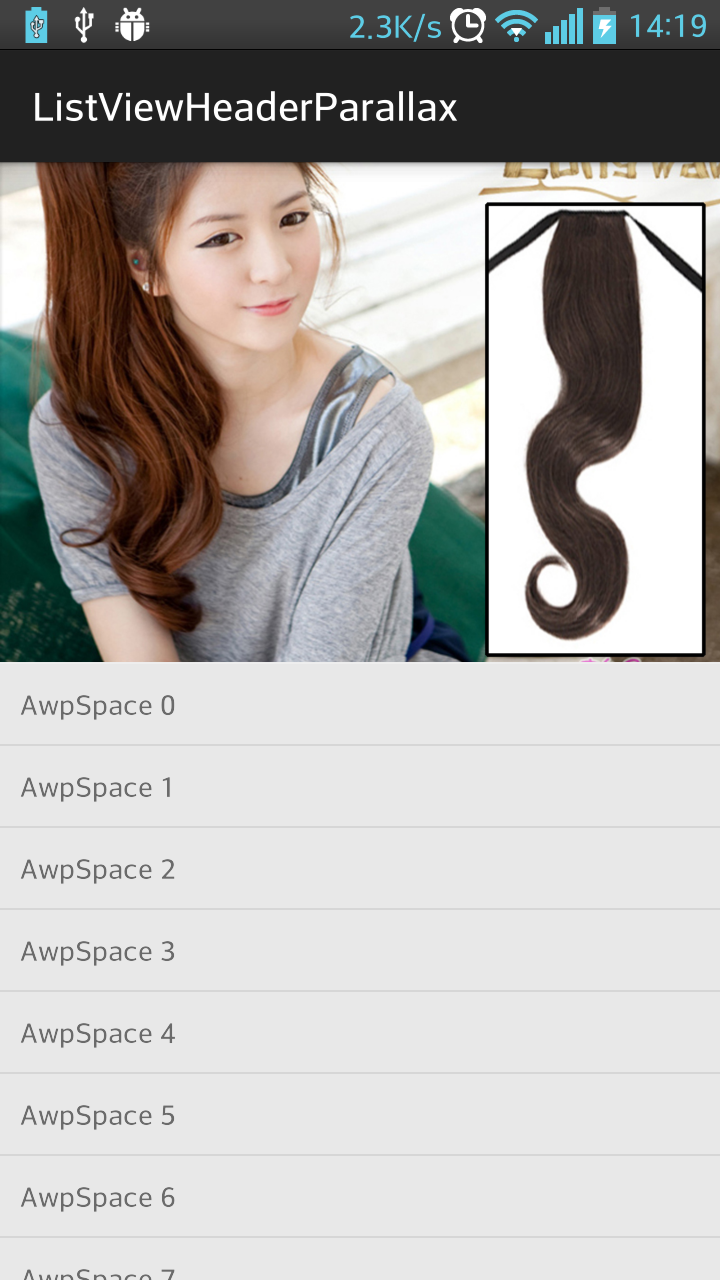
I talked about parallax scrolling, today I will write a post about example to demonstrate ListView Header with parallax effect.
[
If you want to make ListViewHeader with parallax effect, you can follow step by step:
- Create a new android project
- Declare MainActivity layout file (activity_main.xml)
- Declare List header layout file (layout_list_header.xml)
- Declare List row layout file (layout_list_row.xml)
- Implement listview and handle listview scroll events (MainActivity.java)
Create a new android project
We create a new Android project as usual, you can use Eclispe or Android Studio.
Declare MainActivity layout file activity_main.xml
You need add a ListView to the file
<ListView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main_lv_list"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="net.awpspace.listviewheaderparallax.MainActivity" />
Declare List header layout file layout_list_header.xml
You need a image file and copy it to drawable folder. File name is headerimg.jpg. In my example, header’s height is 250dp.
<?xml version="1.0" encoding="utf-8"?> <?xml version="1.0" encoding="utf-8"?>
<FrameLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="250dp"
android:orientation="vertical">
<ImageView android:id="@+id/layout_list_header_iv_image"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"
android:src="@drawable/headerimg" />
</FrameLayout>
Declare List row layout file layout_list_row.xml
<Textview
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#ffe8e8e8"
android:gravity="center_vertical"
android:minheight="40dp"
android:padding="10dp" />
Implement listview and handle listview scroll events (MainActivity.java)
public class MainActivity extends AppCompatActivity implements AbsListView.OnScrollListener {
private static final int SIZE = 50;
private int lastTopValue = 0;
private List < String > mData = new ArrayList < > ();
private ListView mListView;
private ImageView mIvHeader;
private ArrayAdapter mAdapter;
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mListView = (ListView) findViewById(R.id.activity_main_lv_list);
for (int i = 0; i < SIZE; i++) {
mData.add("AwpSpace " + i);
}
mAdapter = new ArrayAdapter(this, R.layout.layout_list_row, mData);
LayoutInflater inflater = getLayoutInflater();
ViewGroup header = (ViewGroup) inflater.inflate(R.layout.layout_list_header, mListView, false);
mListView.addHeaderView(header, null, false);
mListView.setAdapter(mAdapter);
mIvHeader = (ImageView) header.findViewById(R.id.layout_list_header_iv_image);
mListView.setOnScrollListener(this);
}
@Override public void onScrollStateChanged(AbsListView view, int scrollState) { }
@Override public void onScroll(AbsListView view, int firstVisibleItem, int visibleItemCount, int totalItemCount) {
Rect rect = new Rect();
mIvHeader.getLocalVisibleRect(rect);
if (lastTopValue != rect.top) {
lastTopValue = rect.top;
mIvHeader.setY((float)(rect.top / 2.0));
}
}
}
Now, you can run project and see parallax in action.
Download complete source code in here: https://github.com/giolaoit/ListViewHeaderParallax