Implementing Firebase Realtime Database into your Android App
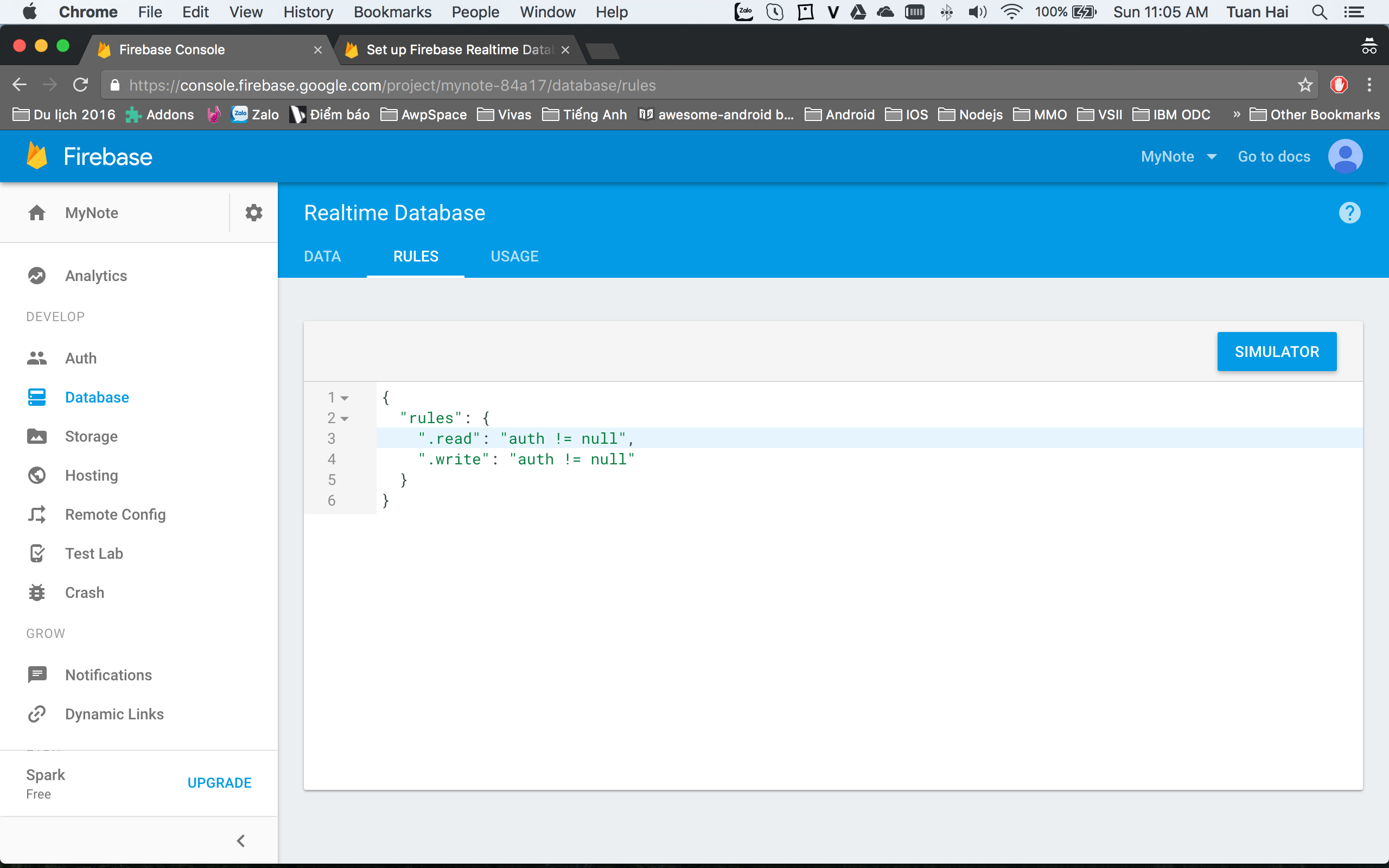
To be continue of the Firebase series, today I will write a new post about Firebase Realtime Database. I have an android application with name is MyNote that is android app allow user can be manage their notes. I had use Firebase Authentication to authenticate user to my app. I will be use Firebase Realtime Database to store user’s data and synchronized in realtime to every connected device.
In this post will be have three main content:
- Introduce to Firebase Realtime Database
- Implementing Firebase Realtime Database to your Android app
- Using Firebase Realtime Database in my app (MyNote)
Introduce to Firebase Realtime Database
Store and sync data with our NoSQL cloud database. Data is synced across all clients in realtime, and remains available when your app goes offline.
The Firebase Realtime Database is a cloud-hosted database. Data is stored as JSON and synchronized in realtime to every connected client. When you build cross-platform apps with our iOS, Android, and JavaScript SDKs, all of your clients share one Realtime Database instance and automatically receive updates with the newest data.
Implementing Firebase Realtime Database to your Android app
Step 1: Connect Firebase to your app
- Install Firebase SDK
- In the Firebase console, add your app to your Firebase project
You can get more detail about how to connect Firebase to your app at my post.
Step 2: Add Realtime Database to your app
Add the dependency for Firebase Realtime Database to your app-level build.gradle file:
compile 'com.google.firebase:firebase-database:9.4.0'
Step 3: Configure Firebase Database Rules
By default, only user authenticated can access to your database. You can get detail about Authentication at my post. But you can configure your rules for public access. Go to Firebase console -> Database -> Rules. You can see default of rules
You can configure your rules for:
- Public: These rules give anyone, even people who are not users of your app, read and write access to your database
{ "rules": { ".read": true, ".write": true } }
- User: These rules grant access to a node matching the authenticated user's ID from the Firebase auth token
{ "rules": { "users": { "$uid": { ".read": "$uid === auth.uid", ".write": "$uid === auth.uid" } } } }
- Private: These rules don't allow anyone read or write access to your database
{ "rules": { ".read": false, ".write": false } }
Step 4: Write data to your database
Retrieve an instance of your database using getInstance()
and reference the location you want to write to.
// Write a message to the database
FirebaseDatabase database = FirebaseDatabase.getInstance();
DatabaseReference myRef = database.getReference("message");
myRef.setValue("Hello, World!");
You can save a range of data types to the database this way, including Java objects. When you save an object the responses from any getters will be saved as children of this location
Step 5: Read from your database
To make your app data update in realtime, you should add a ValueEventListener
to the reference you just created.
The onDataChanged()
method in this class is triggered once when the listener is attached and again every time the data changes, including the children.
// Read from the database
myRef.addValueEventListener(new ValueEventListener() {
@Override public void onDataChange(DataSnapshot dataSnapshot) {
// This method is called once with the initial value and again
// whenever data at this location is updated.
String value = dataSnapshot.getValue(String.class);
Log.d(TAG, "Value is: " + value);
}
@Override public void onCancelled(DatabaseError error) {
// Failed to read value
Log.w(TAG, "Failed to read value.", error.toException());
}
});
Using Firebase Realtime Database in my app (MyNote)
In this app, users can create, delete and synchronize notes between devices.
You can get code for add new note, delete note, fetch notes of user at github.