Material Design Support Library Tutorial - Part 1
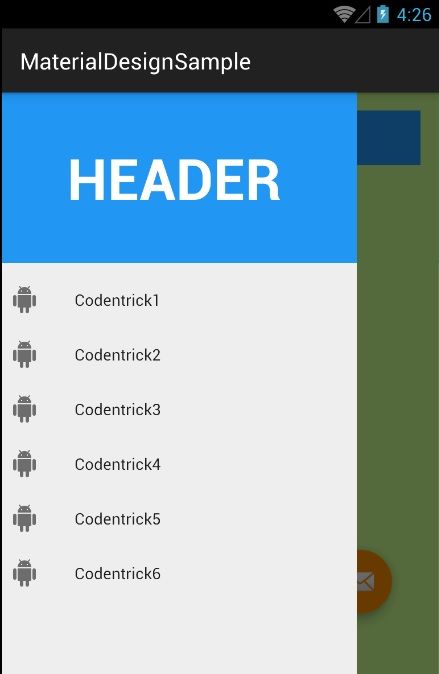
In Google I/O 2015 event, Google announced the support library with named Android Design Support Library providing useful Material Design Components in a single library. Previous to Google announced Android Design Support Library we not easy to implement Material Design in your android application because those Material Design’s UI Component like Floating Action Button wasn’t available in Android pre-Lollipop. Now, we have a bunch of Material Design’s UI Component in Android Design Support Library.
In this article, I show you how to use Android Design Support Library to make some Material Design’s UI components for you application.
About
The demo wil show how to use:
- SnackBar
- Floating Action Button
- EditText Floating Labels
- Navigation View
- Tab Layout
- Coordinator Layout
- ToolBar and CollapsingToolBar Layout
Preparation
Create new Android project with Android Studio
- Applicatiin Name: MaterialDesignSample
- Project Name: android-material-design-support-library-sample
- Package Name: com.codentrick.materialdesignsample
Import Library
- Import android support library v7 appcompat.
- Import android design support library.
- Import cardview library
[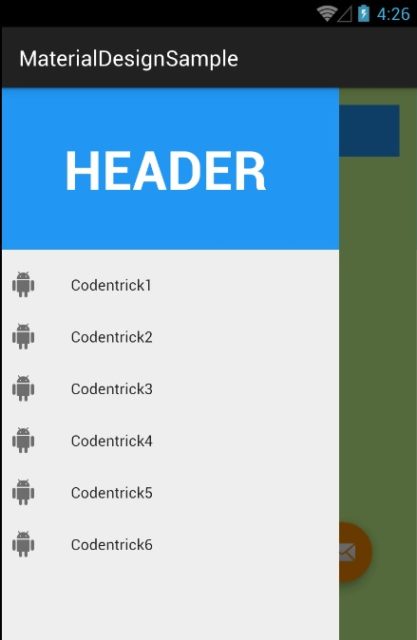](/content/images/2015/08/android_design_support_navigation_view.jpg)