Using Google Firebase in an Android App - Authentication
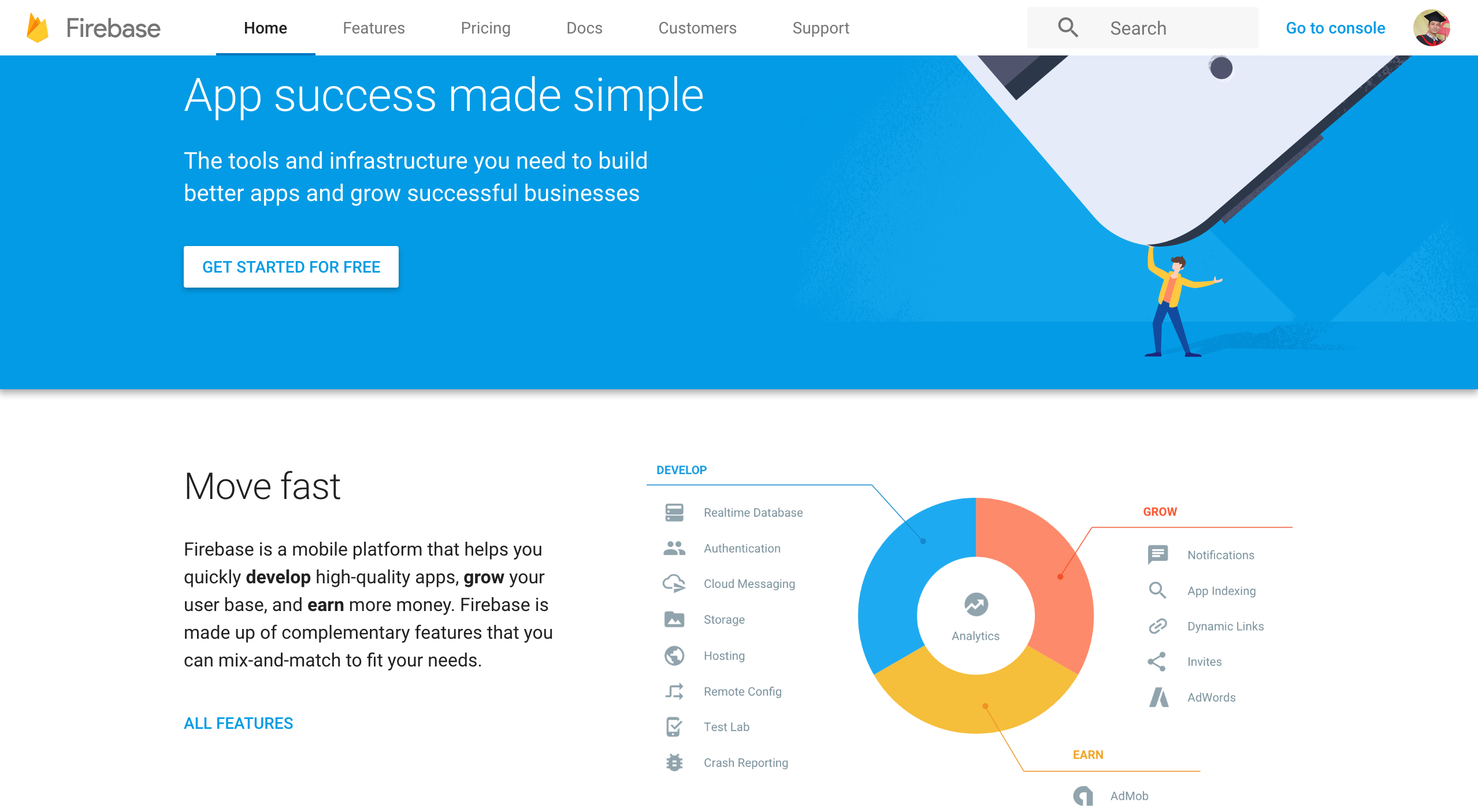
Recently, Google Firebase is a trend of all developers. The new Firebase announcements made at Google I/O 2016 really make Firebase a first-class citizen in the Google ecosystem. Firebase is bringing together all of Google’s best offerings and packaging it into a clean and easy-to-use package.
As an android developer, I want to learn and use Google Firebase to development my android applications. Today I write an article to share to you about how to using Google Firebase in you android app. The first part of series will be talking about using Firebase Auth to authenticating user with email and password.
Create a project on Google Firebase
If you have a Google account you can sign in to Google Firebase and create an project. If you don’t have Google account you must create an account at here. Then you can create a project at Google Firebase.
Add Firebase to android project
Create an android project with Android Studio. You can add Firebase to this project follow by steps.
Step 1: Create android project
Step 2: Add android app on Firebase console
On Firebase console page, you can see like the below
Select “Add Firebase to your Android app”
You must add package name and SHA-1 to this window then click “ADD APP”. Your browser will be auto download a file with name google-services.json
. You follow two steps below to finish.
Enable Auth feature of Google Firebase
On Google Firebase console you select “Auth” tab and then setup Sign-in method with email/password same below.
Add your code for android project
Create three activity on your project and config in manifest.xml file
Check user logged-in. On MainActivity
you can check user logged-in, if user not logged-in my app will start LoginActivity
.
private boolean checkLoggedIn() {
FirebaseUser firebaseUser = FirebaseAuth.getInstance().getCurrentUser();
if (firebaseUser == null) {
return false;
} else {
return true;
}
}
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (!checkLoggedIn()) {
startActivity(new Intent(MainActivity.this, LoginActivity.class));
finish();
}
}
In LoginActivity
you can use SignInWithEmailAndPassword
method to authenticating user.
FirebaseAuth.getInstance().signInWithEmailAndPassword(email, password).addOnCompleteListener(LoginActivity.this, new OnCompleteListener < AuthResult > () {
@Override public void onComplete(@NonNull Task < AuthResult > task) {
if (!task.isSuccessful()) {
Toast.makeText(LoginActivity.this, "Authentication failed.", Toast.LENGTH_SHORT).show();
} else {
startActivity(new Intent(LoginActivity.this, MainActivity.class));
finish();
}
}
});
If user don’t have an account to login, user can open RegisterActivity
to create an account with email and password. To create an account with email android password you can use the method createUserWithEmailAndPassword
.
FirebaseAuth.getInstance().createUserWithEmailAndPassword(email, password).addOnCompleteListener(RegisterActivity.this, new OnCompleteListener < AuthResult > () {
@Override public void onComplete(@NonNull Task < AuthResult > task) {
if (task.isSuccessful()) {
Toast.makeText(RegisterActivity.this, "Register successful!", Toast.LENGTH_SHORT).show();
RegisterActivity.this.finish();
} else {
Toast.makeText(RegisterActivity.this, "Register failed!", Toast.LENGTH_SHORT).show();
}
}
});
When user want to logout you can use method signOut()
FirebaseAuth.getInstance().signOut();
Create an listener to listen state logout successful
private FirebaseAuth.AuthStateListener mAuthListener;
mAuthListener = new FirebaseAuth.AuthStateListener() {
@Override public void onAuthStateChanged(@NonNull FirebaseAuth firebaseAuth) {
FirebaseUser user = firebaseAuth.getCurrentUser();
if (user == null) { // User is signed out startActivity(new Intent(MainActivity.this, LoginActivity.class)); finish(); } } };
@Override public void onStart() {
super.onStart();
FirebaseAuth.getInstance().addAuthStateListener(mAuthListener);
}
@Override public void onStop() {
super.onStop();
if (mAuthListener != null) {
FirebaseAuth.getInstance().removeAuthStateListener(mAuthListener);
}
}
You can get complete code at github!
Below is my app screenshot
When have an user register on your app, you can check on Firebase console same below.
Conclusion
We are finish using Google Firebase to authenticating user with email and password. It very simple but it is a first step to you can be handle power of Google Firebase and apply to your project.
You can get complete code at github!