Implementing Firebase Cloud Messaging (FCM) into your Android App
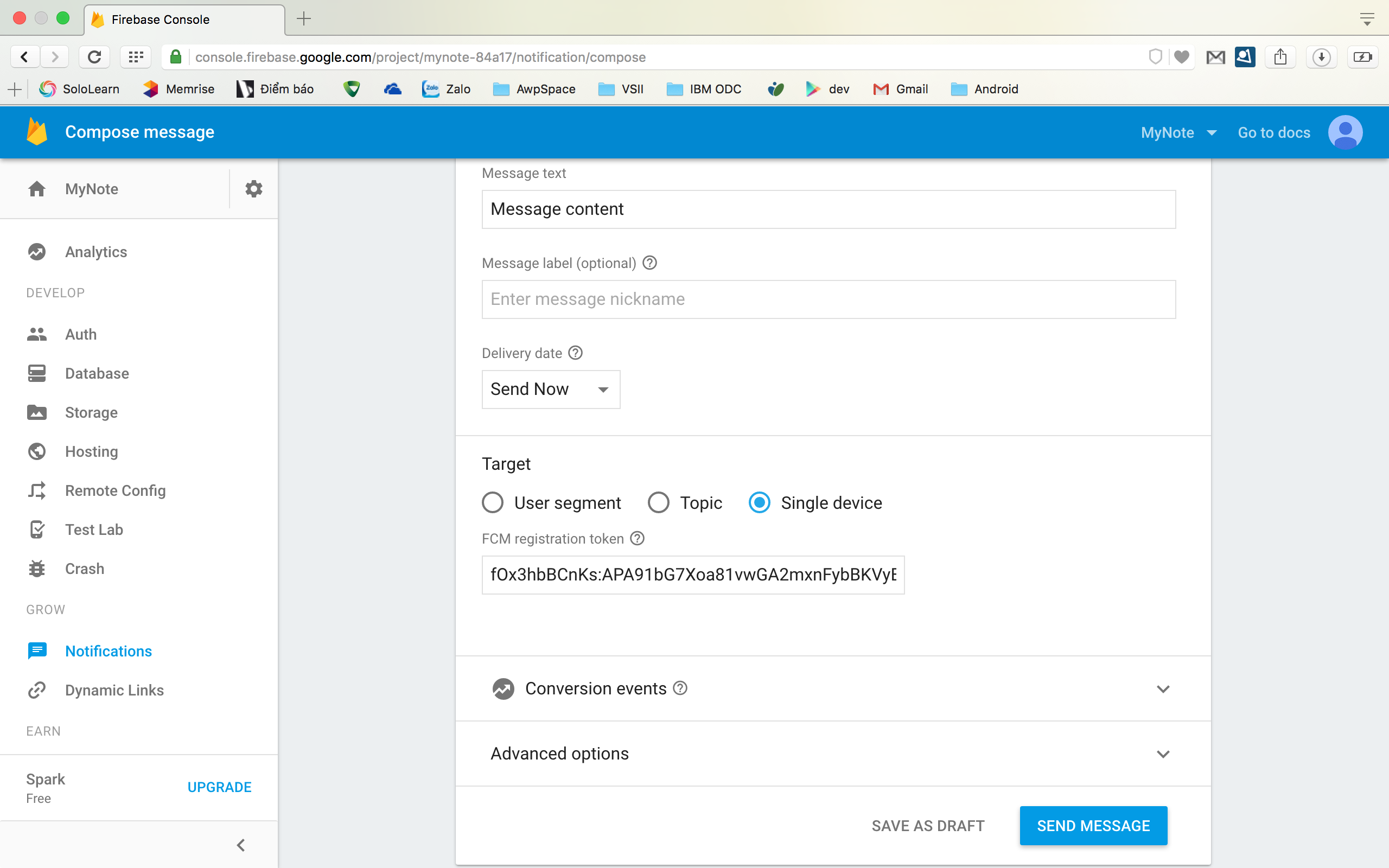
Last month I has an article about Firebase, that content is Firebase Authentication. To continue about topic Google Firebase today I have a new article write about Firebase Cloud Messaging (FCM). I will implement Firebase Cloud Messaging into exist project of the last article. If you don’t know how to creates Firebase project, add Firebase into Android project, you can read at here.
The following steps will provide step by step guide to implement FCM to Android app.
Step 1: Add FCM dependency to your app-level build.gradle
file
dependencies {
compile 'com.google.firebase:firebase-messaging:9.4.0'
}
Step 2: Create services for your app
You must create two services for your app to use FCM. The first service that extends FirebaseMessagingService
. The second service that extends FirebaseInstanceIdService
.
I created two file is MyFirebaseMessagingService and MyFirebaseInstanceIdService.
Step 3: Edit your app manifest
After create service files for your app, you must be add this to your app manifest like following
<service android:name=".fcm.MyFirebaseMessaginService">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/> </intent-filter>
</service>
<service android:name=".fcm.MyFirebaseInstanceIdService">
<intent-filter>
<action android:name="com.google.firebase.INSTANCE_ID_EVENT"/>
</intent-filter>
</service>
Step 4: Retriever the current registration token
When you need to retriever the current token, call
FirebaseInstanceId.getInstance().getToken()
This method will returns null if the token has not yet been generated. Sometime the registration token may changed when:
- The app deletes Instance ID
- The app is restored on a new device
- The user uninstall/reinstall the app
- The user clears app data
In this case you must monitor token generation by method onTokenRefresh that override method in MyFirebaseInstanceIdService.
Step 5: Receive message
You can handling message by method onMessageReceived
that override method in MyFirebaseMessagingService
Step 6: Test notification for your app
Open your project on Google Firebase, you can send a message to your device to test your code.
This article is a basic sample to show to you how to add Google Firebase Cloud Messaging to your Android app. If you have any question you can comment in this post. Thank for read my article and i hope you like it!
You can get complete code at github!